Force





1,554
Rep
Rep
17,181
Likes
Likes
Verified Carder
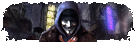
- Posts
- 53,796
- Threads
- 1,598
- Joined
- May 2018
Preauthorization is a means to validate the CC without killing the card. Preauthorization is a means to put a “soft” hold on the CC without actually charging the CC. The Preauth will not show up on the CC statement unless you actually capture the charge within 5-7 days. Otherwise the Preauth hold will expire.
I have written a process using Stripe to Preauth a CC to validate cc number, expiration date and cvv. The process is explained below with the code in PHP. I am assuming you know how to setup a Stripe merchant account. If not then google is your friend.
I have written a process using Stripe to Preauth a CC to validate cc number, expiration date and cvv. The process is explained below with the code in PHP. I am assuming you know how to setup a Stripe merchant account. If not then google is your friend.
PHP:
function createCardToken($number,$month,$year,$cvc){
try {
$token = "";
$token = \Stripe\Token::create(array(
"card" => array(
"number" => $number,
"exp_month" => $month,
"exp_year" => $year,
"cvc" => $cvc
)));
$retval = "success";
return array($retval,$token->id);
} // END TRY
catch (Exception $e) {
$error = $e->getMessage();
$retval = $error;
echo "Token Creation Error: $error <br>";
return array($retval,"");
}
} // End of createCardToken
2. Use the token to Preauth the CC for $1
// Preauthorize Card
function preauthCard($token){
try {
$charge = \Stripe\Charge::create([
'amount' => 100,
'currency' => 'usd',
'description' => 'Preauth charge',
'source' => $token,
'capture' => false,
]);
$retval = "success";
return array($retval,$charge->id);
} // END TRY
catch (Exception $e) {
$error = $e->getMessage();
$retval = $error;
echo "Preauth Error: $error <br>";
return array($retval,"");
}
} // end of Preauth
3. If the Preauth charge is successful then the card is LIVE and CVV is valid. At this point you can either issue a refund or let the Preauth expire on its own.
// Refund the Charge
function createRefund($cid){
try {
$re = \Stripe\Refund::create([
"charge" => $cid ]);
$ref_id = $re->id;
$retval = "success";
return array($retval,$ref_id);
} // END TRY
catch (Exception $e) {
$error = $e->getMessage();
$retval = $error;
echo "Refund Error: $error <br>";
return array($retval,"");
}
} // end of refund charge